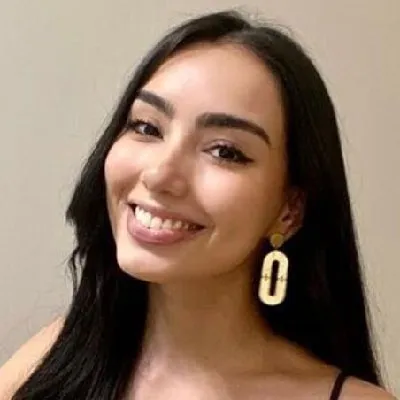
Table of Contents
Want to learn more about this topic? Check out our Office Hours sessions!
Start Using Testkube for Free Today
Testing our cloud native applications is essential to ensure performance, security, and reliability - but doing so correctly represents a huge challenge. If you're working with Go, you must be aware of its highly competent built-in testing functionalities, but it does have a lot of limitations.
A great solution to both these problems comes when putting together Ginkgo and Testkube.
What is Ginkgo Testing?
Ginkgo is a popular, mature, and general purpose testing framework for the Go programming language that, when paired with Gomega, provides a powerful way to write our tests. With Ginkgo, we can run a huge variety of test types in all sorts of contexts: unit tests, integration tests, performance tests, and so on - offering a simple yet powerful solution to most of our testing needs… but, how can we translate these tests into cloud native applications? With Testkube, this is no longer a challenge.
Testkube is Kubernetes-native testing framework for testers and developers. It acts as an executor so you can orchestrate, run, and display tests and test results for your code in a Kubernetes environment. With out-of-the-box support for multiple and popular testing tools, Testkube simplifies the transition into a Kubernetes environment so you can truly focus on testing.
In this tutorial, I'll walk you through how this integration works and a couple examples so you can get your Go lang testing Go-ing with Ginkgo. 😉
How can Ginkgo be used for cloud native testing?
Testkube supports a great variety of testing tools and frameworks, including Ginkgo. How is this done?
With Testkube, each of the supported testing tools is defined as a CRD (Kubernetes Custom Resource) and called an Executor. An Executor is nothing more than a wrapper around a testing framework in the shape of a Docker container, which is then run as a Kubernetes job.
When you install Testkube, you have native access to a great variety of tools that we've gone ahead and implemented for you. Here's how we defined it for none other than our Ginkgo executor:
```yaml
apiVersion: executor.testkube.io/v1
kind: Executor
metadata:
name: ginkgo-executor
namespace: testkube
spec:
features:
- artifacts
- junit-report
image: kubeshop/testkube-ginkgo-executor:0.0.4
types:
- ginkgo/test
```
Now that we know what's happening behind the scenes - let's go ahead and install Testkube!
Installing Testkube
To install Testkube, you'll need to have a cluster up and running. Then, there's two ways you can handle the installation:
Installing Testkube with helm:
```bash
helm repo add testkube https://kubeshop.github.io/helm-charts && \
helm repo update && \
helm install --create-namespace my-testkube testkube/testkube --namespace testkube
```
Install Testkube through the CLI:
On MacOS
```bash
brew install testkube
```
On Ubuntu/Debian
```bash
wget -qO - https://repo.testkube.io/key.pub | sudo apt-key add - && echo "deb https://repo.testkube.io/linux linux main" | sudo tee -a /etc/apt/sources.list && sudo apt-get update && sudo apt-get install -y testkube
```
On Windows
```bash
choco source add --name=kubeshop_repo --source=https://chocolatey.kubeshop.io/chocolatey
choco install testkube -y
```
After successfully installing Testkube, let's initiate it within our cluster to install all the necessary components. For this, simply type in the following command:
```bash
testkube init
```
And that's it! Let's get started with our Ginkgo tests.
Writing Ginkgo Golang Tests
Like we mentioned before, Ginkgo allows us to write multiple types of tests. Built on top of Go's testing infrastructure, it lets developers and testers write more expressive tests by using the blocks `Describe`, `Context`, `It`, `BeforeEach`, and `AfterEach`. These let us define what we're testing, when, and before or after certain unit tests.
For the purpose of this tutorial, we'll start with a simple one to test an HTTP request and response. For this, we'll install Ginkgo and bootstrap our project:
```bash
ginkgo bootstrap
```
We'll create a simple test called `url_test.go`:
```Go
package example
import (
"net/http"
. "github.com/onsi/ginkgo/v2"
. "github.com/onsi/gomega"
)
var _ = Describe("URL Test", func() {
It("should visit testkube.io and return 200", func() {
resp, err := http.Get("https://testkube.io")
Expect(err).To(BeNil())
Expect(resp.StatusCode).To(Equal(200))
})
})
```
Simultaneously, we'll create another test that checks the entries for an endpoint. We'll call it `api_test.go`:
```Go
package example
import (
"encoding/json"
"io"
"net/http"
. "github.com/onsi/ginkgo/v2"
. "github.com/onsi/gomega"
)
var _ = Describe("API Test", func() {
It("There should be user entries", func() {
resp, err := http.Get("https://jsonplaceholder.typicode.com/users")
Expect(err).To(BeNil())
body, err := io.ReadAll(resp.Body)
Expect(err).To(BeNil())
var data []map[string]interface{}
err = json.Unmarshal(body, &data)
Expect(err).To(BeNil())
Expect(len(data)).To(BeNumerically(">", 0))
})
})
```
Now, let's make sure that our tests run locally. This should be our expected output:
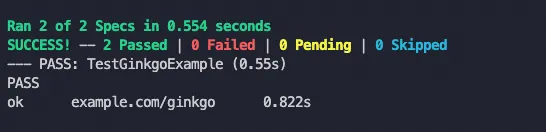
Success! Now let's push these onto our cluster. Since Ginkgo projects are usually more verbose, we'll push our project to GitHub and reference it when running it on Testkube. You can find the project here.
Running Ginkgo Tests in Kubernetes With Testkube
Whether you're a terminal or UI person, there's two ways we can go ahead and create our tests.
To launch the UI, type in the following command:
```bash
testkube dashboard
```
This will launch our UI on your browser. Once there, let's click on Add a new test:
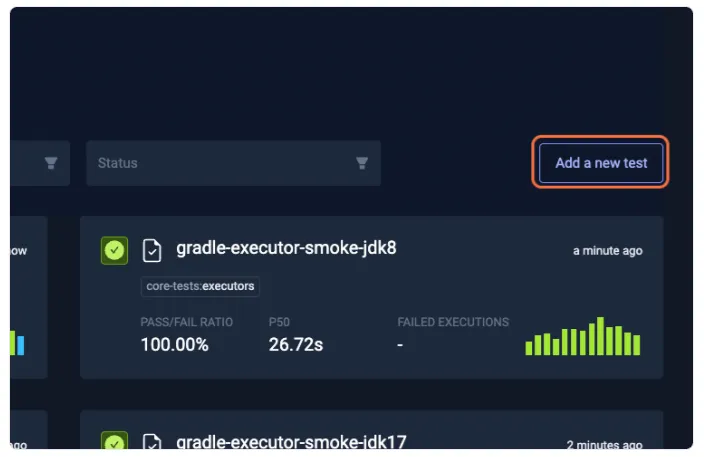
Assign a name to our test:
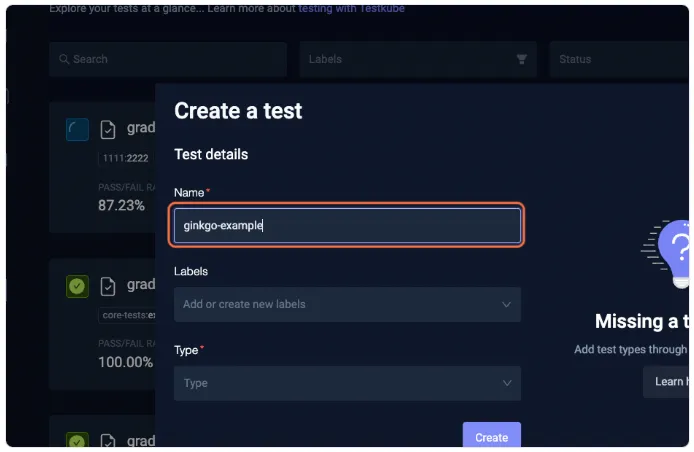
We'll call it `ginkgo-example`.
And select the ginkgo/test type in the Type drop-down menu:
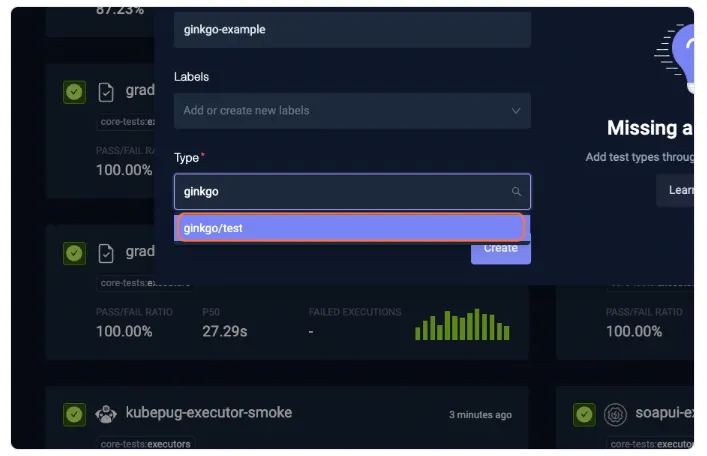
Now, we'll tell Testkube how to get our test files. In this case, we'll reference the git repository we just created:

Paste our git URI:

Select the main branch:

And click on Create.
Alternatively, you can create your test straight from Testkube's CLI by typing the following command:
```bash
kubectl testkube create test --git-uri https://github.com/alelthomas/ginkgo-example.git --git-path examples/testkube-api --type ginkgo/test --name ginkgo-example-test --git-branch main
```
Now that our test is created, let's go and run it!
On the dashboard, click on Run now.

Or, run it from your terminal with the the following command:
```bash
kubectl testkube run test ginkgo-example
```
Our test is now running!
On the dashboard, you can see the logs in present time by clicking on your test's execution:
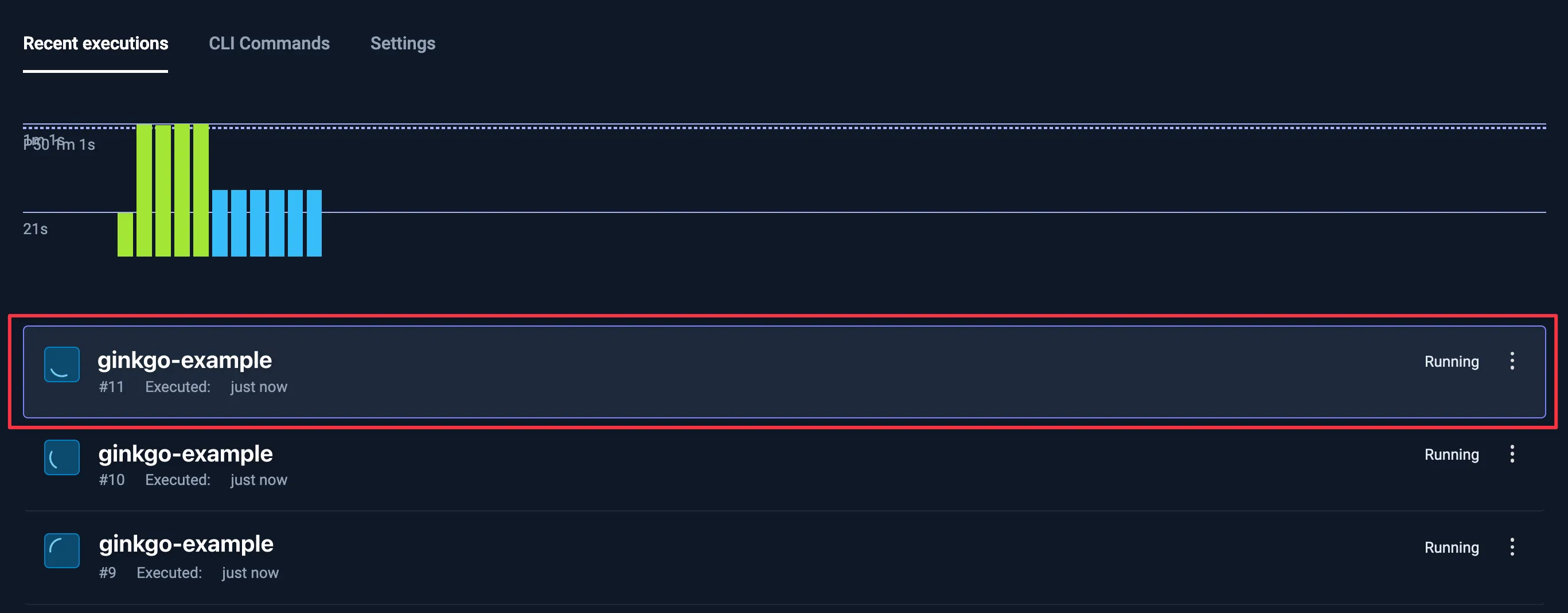
In the terminal, you can follow the execution by typing in the following:
```bash
kubectl testkube get execution [execution-number]
```
And that's it! You can see that our tests ran and passed successfully without any hassling configurations:
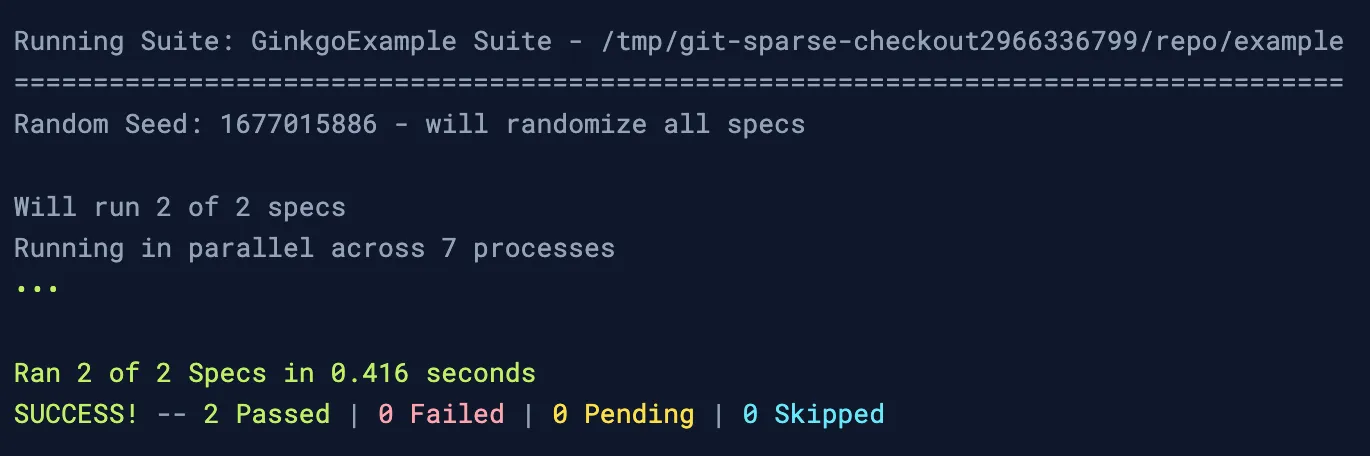
By simply having your tests written, Testkube grabs them and executes them separately so you don't have to worry about the noise.
Conclusion
As you can see, although Ginkgo can grant us much more depth for creating expressive tests with Go, executing them in Kubernetes doesn't have to be painful.
In this tutorial, I showed you two very simple tests, but the possibilities are endless and can help you in truly ensuring that your apps remain stable and reliable.
You can check out more Ginkgo example projects here or visit our documentation page for our Ginkgo executor.
Give it a go!
Why not give it a go yourself? Sign up to Testkube and try one of our examples or head over to our documentation - if you get stuck or have questions, we’re here to help! Find an answer to your questions in the Testkube Knowledge Base or reach out to us on Slack. We’re eager to hear how you use our integrations!