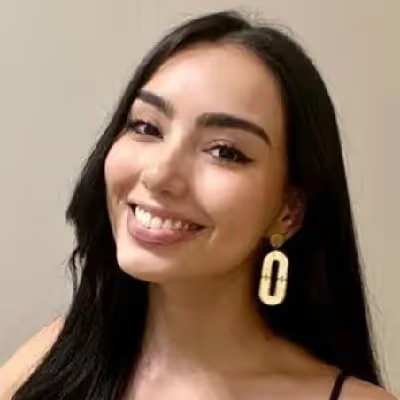
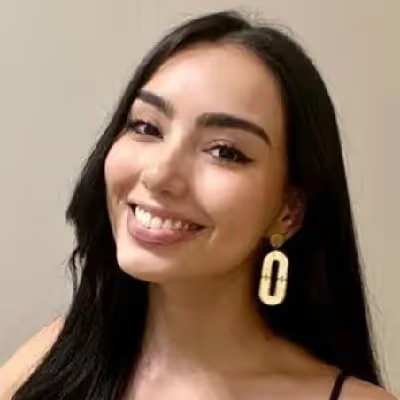
Table of Contents
Want a Personalized Feature Set Demo?
Unlock Better Testing Workflows in Kubernetes — Try Testkube for Free
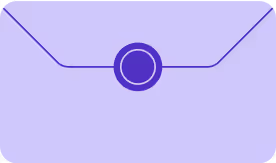

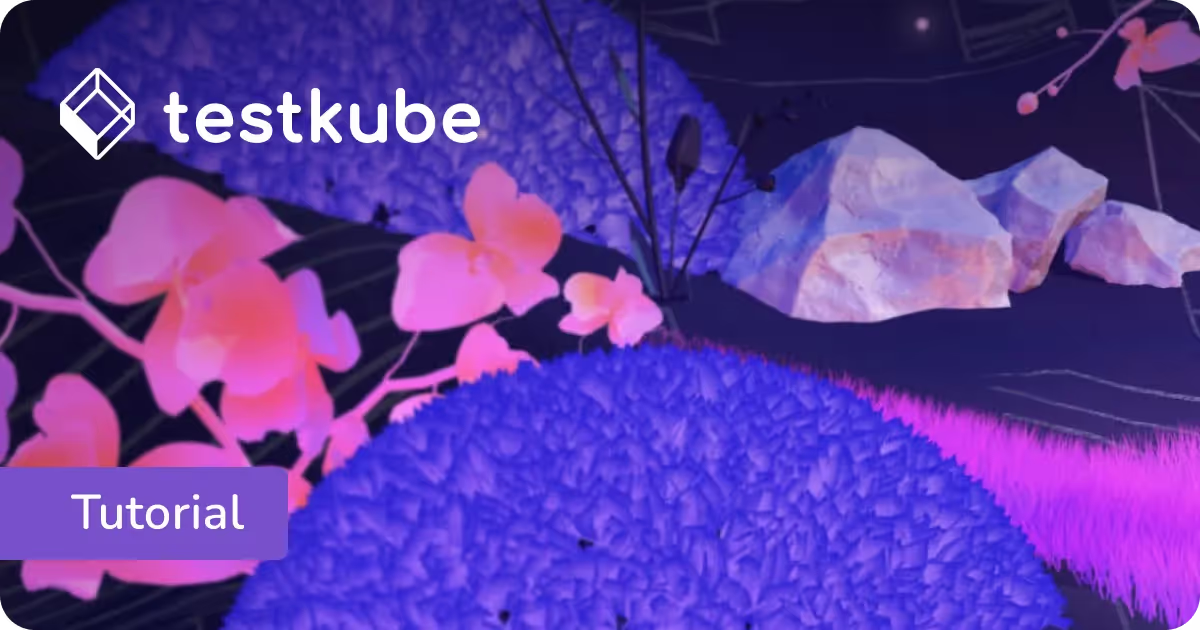
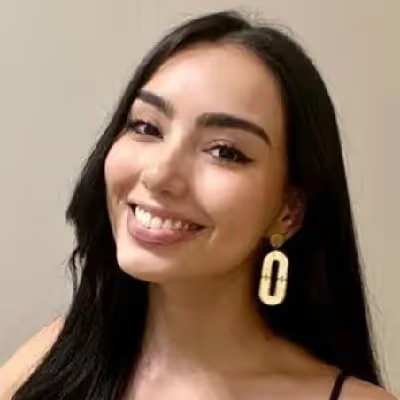
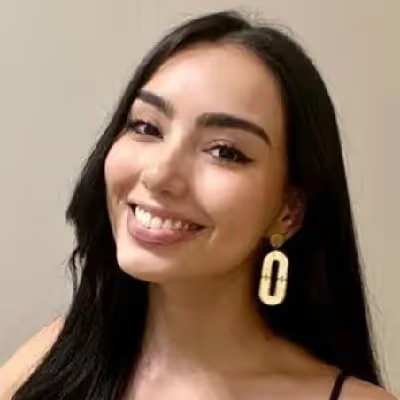
Table of Contents
The Java world is huge and complicated on its own – and if you add Kubernetes to the mix, the complexity levels can skyrocket for most software engineers. Those who want to use Java tests for Java projects often struggle with running the tests in Kubernetes as it's a very complicated, pluggable environment with tons of constraints and many configuration options.
Testkube is a Kubernetes-native testing framework that allows you to orchestrate, execute, and run all your tests in a cluster while providing you with a common view of your system behaviour. To allow for the integration of Java-based tests, we added the ability to run tests on one of the most popular build tools from the Java ecosystem: Gradle.
This integration allows us to define and run any Java-based test case, and you can also choose a particular Java version either for Maven or Gradle test executors - meaning that our build pipelines are building Docker images against the most popular Java versions.
In this article, we’ll walk you through integrating Gradle and Maven to run your tests using Testkube.
Running REST Assured with Gradle
Let's get to work with Gradle first! For the purpose of this tutorial, we’ll work with a project based on the REST Assured testing framework.
Let’s start by writing up our Java-based test first. Using Testkube’s Labels feature, we’ll need to write the test around Labels and Test Execution endpoints: so we’ll use Testkube API, which is based on OpenAPI spec for that particular task.
We’ll write a couple tests that will simply check for labels and executions, as shown below:
package testkube;
import org.junit.jupiter.api.Test;
import static io.restassured.RestAssured.*;
import static org.hamcrest.Matchers.*;
public class TestkubeAPITest {
private static final String apiURI = "https://demo.testkube.io/results/v1";
@Test
void hasAtLeastOneLabel() {
given()
.baseUri(apiURI)
.when()
.get("/labels")
.then()
.assertThat()
.body("app.size()", greaterThan(1))
;
}
@Test
void hasSomeExecutions() {
given()
.baseUri(apiURI)
.when()
.get("/executions")
.then()
.assertThat()
.body("results.size()", greaterThan(1))
.body("totals.results", greaterThan(1))
;
}
}
All examples can be found in our Testkube blog resources repository in gradle-restassured
subdirectory.
Now that our test is ready to go, we’ll need to have Testkube installed in our Kubernetes cluster and create a new Test resource with the kubectl testkube
plugin:
kubectl testkube create test --git-uri https://github.com/kubeshop/testkube-blog-resources.git --git-path gradle-restassured --type gradle/test --name testkube-api-restassured --git-branch main
Now let’s run our test:
kubectl testkube run test testkube-api-restassured -f
Then after the execution, we can see that our tests succeeded:
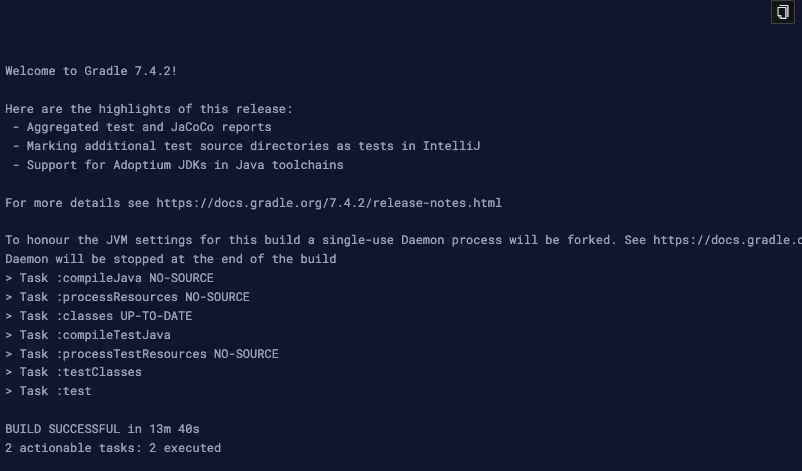
To make sure that it’s doing what it’s supposed to, let’s change the test to make it fail:
@Test
void apiIsBlazinglyFast() {
given()
.baseUri(apiURI)
.when()
.get("/executions")
.then()
.assertThat()
.time(lessThan(10L))
;
}
This test is meant to fail using our demo, since this particular endpoint is way slower than 10ms. Now, since our test definition was based on Git, we’ll just push our changes to Git and run the same Testkube test again.
Note: We’ve defined our test based on a Git repo, but we could also store simple test data in our Test Custom Resource as a string (like with Postman collections that are a single JSON file). The reason why our test is saved in a Git repo is so we can run it again and validate if it’s failing.
After updating our repo, let’s run our test again:
kubectl testkube run test testkube-api-restassured -f
We can see that our out of our 3 tests, the one we recently added failed:

Cool! Looks like we’re on the right track.
Now, onto the next issue: we’ve just run our test against a JDK 11-based Gradle build (our default). But what if our tests had constraints around a Java version? Testkube can also help us here!
Instead of managing Java versions manually or messing around with Docker containers with all the possible JDKs, we’ll simply create an additional Executor based on JDK 18.
Let’s create our new Gradle executor as a gradle-jdk18-executor.yaml file:
apiVersion: executor.testkube.io/v1
kind: Executor
metadata:
annotations:
name: gradle-jdk18-executor
namespace: testkube
spec:
image: kubeshop/testkube-gradle-executor:0.1.4-jdk18
types:
- gradle:jdk18/project
- gradle:jdk18/test
- gradle:jdk18/integrationTest
Note: types: gradle:jdk18
is our convention around creating new Kubernetes Kinds and coupling tests together.
Let’s kubeclt apply our file, and allow Kubernetes to create a new resource for us.
sh kubectl apply -f gradle-jdk18-executor.yaml
When an executor is created, we can create a test based on our new test type handled by the new executor:
kubectl testkube create test --git-uri https://github.com/kubeshop/testkube-blog-resources.git --git-path gradle-restassured --type gradle<strong>:jdk18</strong>/test --name testkube-api-restassured --git-branch main
Notice the :jdk18
suffix? We’ve just created the same test handled by a different executor - this is possible thanks to one of Testkube’s many features!
P.S. Testkube can also handle Kotlin based .kts
Gradle build files.
This is all pretty neat, but what if you’re using Maven instead? Don’t worry - Testkube can also handle Maven projects!
Running REST Assured with Maven
Let’s start by creating a new Maven test. We’ll assume the same test class, but using our different build tool.
Let’s go back to what our test looks like:
public class TestkubeAPITest {
private static final String apiURI = "https://demo.testkube.io/results/v1";
@Test
void hasAtLeastOneLabel() {
given()
.baseUri(apiURI)
.when()
.get("/labels")
.then()
.assertThat()
.body("app.size()", greaterThan(1))
;
}
@Test
void hasSomeExecutions() {
given()
.baseUri(apiURI)
.when()
.get("/executions")
.then()
.assertThat()
.body("results.size()", greaterThan(1))
.body("totals.results", greaterThan(1))
;
}
}
Let’s create some standard Maven pom.xml
files and create a new Maven test in Testkube.
You can find the code for this here.
Now, let’s create our Test:
kubectl testkube create test --git-uri https://github.com/kubeshop/testkube-blog-resources.git --git-path maven-restassured --type maven/test --name testkube-api-restassured-maven --git-branch main
And run it:
kubectl testkube run test testkube-api-restassured-maven -f
After many, many lines of logs, we can see that our build was successful!

NOTE: If you need to use wrappers (mvnw, gradlew) just put them side-by-side to build.gradle or pom.xml files, we’ll also handle that for you.
Conclusion
Running Java based tests in Kubernetes doesn't need to be hard. Luckily for us, Testkube can help.
For this example, we just ran simple tests on external services, but what if we wanted to test an internal service without an Ingress or LoadBalancers? It would be quite complicated to do it from your CI/CD platform, but Testkube can help as its runners are just native Kubernetes jobs that are running inside the cluster.
Why not give it a go yourself? Sign up to Testkube and try one of our examples or head over to our documentation - if you get stuck—we’re here to help! Reach out to us on Slack. We’re eager to hear how you use our integrations.
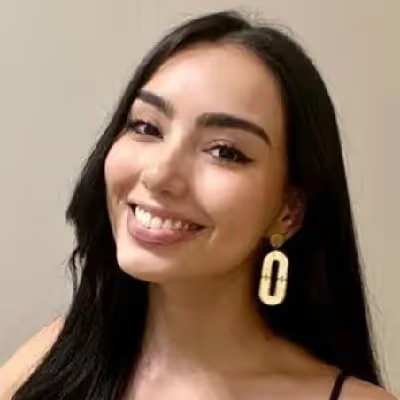
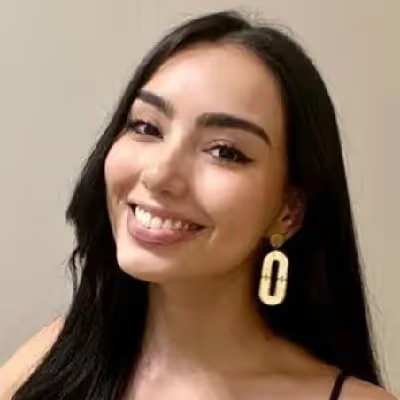